Read Only Collections
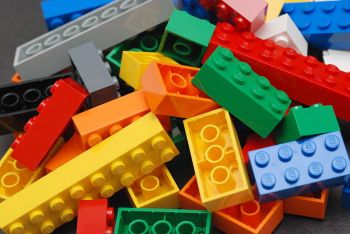
But this is not the case in programming. You create a java collection and store objects in it. Then there are scenarios where you want them not be modified. Obsessed with file system terminology Java guys have named it as read only collections.
By default some of the languages like dot net provide read only collections. But in Java there are no such things. This is not a special type of collection it is an additional facility provided to change the usual collections as read only.
Methods by Collections class
The Collections class provides six factory methods, one for each of Collection, List, Map, Set, SortedMap, and SortedSet.- Collection unmodifiableCollection(Collection collection)
- List unmodifiableList(List list)
- Map unmodifiableMap(Map map)
- Set unmodifiableSet(Set set)
- SortedMap unmodifiableSortedMap(SortedMap map)
- SortedSet unmodifiableSortedSet(SortedSet set)
The returned set will be serializable if the specified set is serializable. If you attempt to modify a read-only collection it will throw an UnsupportedOperationException.
Example source code for java read only collections
import java.util.ArrayList; |
import java.util.Arrays; |
import java.util.Collections; |
import java.util.HashMap; |
import java.util.HashSet; |
import java.util.List; |
import java.util.Map; |
import java.util.Set; |
// example program to demonstrate the read-only collection in java |
public class ReadOnlyCollections { |
public static void main(String args[]) { |
// creating a list |
List godList = Arrays |
.asList( new String[] { "Donald" , "Dennis" , "Ken" }); |
// making a read-only list |
List list = new ArrayList(godList); |
list = Collections.unmodifiableList(list); |
// checking the reference in a read-only set |
Set set = new HashSet(godList); |
Collections.unmodifiableSet(set); |
// the following statement allows to modify the above set as the |
// reference is pointing to the original collection therefore it is not |
// read-only |
set.add( "Alan" ); |
// making a read-only map and try to modify it |
Map godMap = new HashMap(); |
godMap.put( "TAOCP" , "Donald" ); |
godMap.put( "C" , "Dennis" ); |
godMap = Collections.unmodifiableMap(godMap); |
try { |
// modifying the read-only map to check what happens |
godMap.put( "Unix" , "Ken" ); |
} catch (UnsupportedOperationException e) { |
System.out.println( "You cannot modify a read only collection!" ); |
} |
} |
}
Difference between Vector and ArrayList in java?
All the methods of Vector is synchronized. But, the methods of ArrayList is not synchronized. All the new implementations of java collection framework is not synchronized.
Vector and ArrayList both uses Array internally as data structure. They are dynamically resizable. Difference is in the way they are internally resized. By default, Vector doubles the size of its array when its size is increased. But, ArrayList increases by half of its size when its size is increased.
Therefore as per Java API the only main difference is, Vector’s methods are synchronized and ArrayList’s methods are not synchronized.
Vector or ArrayList? Which is better to use in java?
In general, executing a ‘synchronized’ method results in costlier performance than a unsynchronized method. Keeping the difference in mind, using Vector will incur a performance hit than the ArrayList. But, when there is a certain need for thread-safe operation Vector needs to be used.
Is there an alternate available in java for Vector?
ArrayList can be synchronized using the java collections framework utility class and then ArrayList itself can be used in place of Vector.
When there is no need for synchronized operation and you still look for better performance ‘Array’ can be used instead of ArrayList. But the development is tedious, since it doesn’t provide user friendly methods.
When you use Vector or ArrayList, always initialize to the largest capacity that the java program will need. Since incrementing the size is a costlier operation.
Session Tracking Methods
What is a session?
A session is a conversation between the server and a client. A conversation consists series of continuous request and response.Why should a session be maintained?
When there is a series of continuous request and response from a same client to a server, the server cannot identify from which client it is getting requests. Because HTTP is a stateless protocol.When there is a need to maintain the conversational state, session tracking is needed. For example, in a shopping cart application a client keeps on adding items into his cart using multiple requests. When every request is made, the server should identify in which client’s cart the item is to be added. So in this scenario, there is a certain need for session tracking.
Solution is, when a client makes a request it should introduce itself by providing unique identifier every time. There are five different methods to achieve this.
Session tracking methods:
- User authorization
- Hidden fields
- URL rewriting
- Cookies
- Session tracking API
1. User Authorization
Users can be authorized to use the web application in different ways. Basic concept is that the user will provide username and password to login to the application. Based on that the user can be identified and the session can be maintained.2. Hidden Fields
<INPUT TYPE=”hidden” NAME=”technology” VALUE=”servlet”>Hidden fields like the above can be inserted in the webpages and information can be sent to the server for session tracking. These fields are not visible directly to the user, but can be viewed using view source option from the browsers. This type doesn’t need any special configuration from the browser of server and by default available to use for session tracking. This cannot be used for session tracking when the conversation included static resources lik html pages.
3. URL Rewriting
Original URL: http://server:port/servlet/ServletNameRewritten URL: http://server:port/servlet/ServletName?sessionid=7456
When a request is made, additional parameter is appended with the url. In general added additional parameter will be sessionid or sometimes the userid. It will suffice to track the session. This type of session tracking doesn’t need any special support from the browser. Disadvantage is, implementing this type of session tracking is tedious. We need to keep track of the parameter as a chain link until the conversation completes and also should make sure that, the parameter doesn’t clash with other application parameters.
4. Cookies
Cookies are the mostly used technology for session tracking. Cookie is a key value pair of information, sent by the server to the browser. This should be saved by the browser in its space in the client computer. Whenever the browser sends a request to that server it sends the cookie along with it. Then the server can identify the client using the cookie.In java, following is the source code snippet to create a cookie:
Cookie cookie = new Cookie(“userID”, “7456″);
res.addCookie(cookie);
Session tracking is easy to implement and maintain using the cookies. Disadvantage is that, the users can opt to disable cookies using their browser preferences. In such case, the browser will not save the cookie at client computer and session tracking fails.
5. Session tracking API
Session tracking API is built on top of the first four methods. This is inorder to help the developer to minimize the overhead of session tracking. This type of session tracking is provided by the underlying technology. Lets take the java servlet example. Then, the servlet container manages the session tracking task and the user need not do it explicitly using the java servlets. This is the best of all methods, because all the management and errors related to session tracking will be taken care of by the container itself.Every client of the server will be mapped with a javax.servlet.http.HttpSession object. Java servlets can use the session object to store and retrieve java objects across the session. Session tracking is at the best when it is implemented using session tracking api.
Session Tracking Methods
What is a session?
A session is a conversation between the server and a client. A conversation consists series of continuous request and response.Why should a session be maintained?
When there is a series of continuous request and response from a same client to a server, the server cannot identify from which client it is getting requests. Because HTTP is a stateless protocol.When there is a need to maintain the conversational state, session tracking is needed. For example, in a shopping cart application a client keeps on adding items into his cart using multiple requests. When every request is made, the server should identify in which client’s cart the item is to be added. So in this scenario, there is a certain need for session tracking.
Solution is, when a client makes a request it should introduce itself by providing unique identifier every time. There are five different methods to achieve this.
Session tracking methods:
- User authorization
- Hidden fields
- URL rewriting
- Cookies
- Session tracking API
1. User Authorization
Users can be authorized to use the web application in different ways. Basic concept is that the user will provide username and password to login to the application. Based on that the user can be identified and the session can be maintained.2. Hidden Fields
<INPUT TYPE=”hidden” NAME=”technology” VALUE=”servlet”>Hidden fields like the above can be inserted in the webpages and information can be sent to the server for session tracking. These fields are not visible directly to the user, but can be viewed using view source option from the browsers. This type doesn’t need any special configuration from the browser of server and by default available to use for session tracking. This cannot be used for session tracking when the conversation included static resources lik html pages.
3. URL Rewriting
Original URL: http://server:port/servlet/ServletNameRewritten URL: http://server:port/servlet/ServletName?sessionid=7456
When a request is made, additional parameter is appended with the url. In general added additional parameter will be sessionid or sometimes the userid. It will suffice to track the session. This type of session tracking doesn’t need any special support from the browser. Disadvantage is, implementing this type of session tracking is tedious. We need to keep track of the parameter as a chain link until the conversation completes and also should make sure that, the parameter doesn’t clash with other application parameters.
4. Cookies
Cookies are the mostly used technology for session tracking. Cookie is a key value pair of information, sent by the server to the browser. This should be saved by the browser in its space in the client computer. Whenever the browser sends a request to that server it sends the cookie along with it. Then the server can identify the client using the cookie.In java, following is the source code snippet to create a cookie:
Cookie cookie = new Cookie(“userID”, “7456″);
res.addCookie(cookie);
Session tracking is easy to implement and maintain using the cookies. Disadvantage is that, the users can opt to disable cookies using their browser preferences. In such case, the browser will not save the cookie at client computer and session tracking fails.
5. Session tracking API
Session tracking API is built on top of the first four methods. This is inorder to help the developer to minimize the overhead of session tracking. This type of session tracking is provided by the underlying technology. Lets take the java servlet example. Then, the servlet container manages the session tracking task and the user need not do it explicitly using the java servlets. This is the best of all methods, because all the management and errors related to session tracking will be taken care of by the container itself.Every client of the server will be mapped with a javax.servlet.http.HttpSession object. Java servlets can use the session object to store and retrieve java objects across the session. Session tracking is at the best when it is implemented using session tracking api.
What happens if you call destroy() from init() in java servlet?
In java servlet, destroy() is not supposed to be called by the programmer. But, if it is invoked, it gets executed. The implicit question is, will the servlet get destroyed? No, it will not. destroy() method is not supposed to and will not destroy a java servlet. Don’t get confused by the name. It should have been better, if it was named onDestroy().
The meaning of destroy() in java servlet is, the content gets executed just before when the container decides to destroy the servlet. But if you invoke the destroy() method yourself, the content just gets executed and then the respective process continues. With respective to this question, the destroy() gets executed and then the servlet initialization gets completed.
Have a look at this java servlet interview question: Servlet Life Cycle – Explain, it might help you to understand better.
How to avoid IllegalStateException in java servlet?
It is always better to ensure that no content is added to the response after the forward or redirect is done to avoid IllegalStateException. It can be done by including a ‘return’ statement immediately next to the forward or redirect statement.
Example servlet source code snippet:
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
if("success".equals(processLogin())) {
response.sendRedirect("menu.jsp");
return; // <-- this return statement ensures that no content is adedd to the response further
}
Note: This same scenario of IllegalStateException is applicable in JSP also.
/*
other servlet code that may add to the response….
*/
}
What is servlet mapping?
How is servlet mapping defined?
Servlets should be registered with servlet container. For that, you should add entries in web deployment descriptor web.xml. It is located in WEB-INF directory of the web application.Entries to be done in web.xml for servlet-mapping:
<servlet-mapping>
<servlet-name>milk</servlet-name>
<url-pattern>/drink/*</url-pattern>
</servlet-mapping>
servlet-mapping has two child tags, url-pattern and servlet-name. url-pattern specifies the type of urls for which, the servlet given in servlet-name should be called. Be aware that, the container will use case-sensitive for string comparisons for servlet matching.
Syntax for servlet mapping as per servlet specification SRV.11.2:
A string beginning with a ‘/’ character and ending with a ‘/*’ suffix is used for path mapping.A string beginning with a ‘*.’ prefix is used as an extension mapping.
A string containing only the ‘/’ character indicates the “default” servlet of the application. In this case the servlet path is the request URI minus the context path and the path info is null.
All other strings are used for exact matches only.
Rule for URL path mapping:
It is used in the following order. First successful match is used with no further attempts.1. The container will try to find an exact match of the path of the request to the path of the servlet. A successful match selects the servlet.
2. The container will recursively try to match the longest path-prefix. This is done by stepping down the path tree a directory at a time, using the ’/’ character as a path separator. The longest match determines the servlet selected.
3. If the last segment in the URL path contains an extension (e.g. .jsp), the servlet container will try to match a servlet that handles requests for the extension. An extension is defined as the part of the last segment after the last ’.’ character.
4. If neither of the previous three rules result in a servlet match, the container will attempt to serve content appropriate for the resource requested. If a “default” servlet is defined for the application, it will be used.
What is implicit mapping?
A servlet container can have a internal JSP container. In such case, *.jsp extension is mapped to the internal container. This mapping is called implicit mapping. This implicit mapping allows ondemand execution of JSP pages. Servlt mapping defined in web application has high precedence over the implicit mapping.Example code for java servlet mapping:
<servlet><servlet-name>milk</servlet-name>
<servlet-class>com.javapapers.Milk</servlet-class>
</servlet>
<servlet>
<servlet-name>points</servlet-name>
<servlet-class>com.javapapers.Points</servlet-class>
</servlet>
<servlet>
<servlet-name>controller</servlet-name>
<servlet-class>com.javapapers.ControllerServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>milk</servlet-name>
<url-pattern>/drink/*</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>points</servlet-name>
<url-pattern>/pointlist</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>controller</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
What is Servlet Invoker?
As defined by Apache Tomcat specification, the purpose of Invoker Servlet is to allow a web application to dynamically register new servlet definitions that correspond with a <servlet> element in the /WEB-INF/web.xml deployment descriptor.By enabling servlet invoker the servlet mapping need not be specified for servlets. Servlet ‘invoker’ is used to dispatch servlets by class name.Enabling the servlet invoker can create a security hole in web application. Because, Any servlet in classpath even also inside a .jar could be invoked directly. The application will also become not portable. Still if you want to enable the servlet invoker consult the web server documentation, because every server has a different method to do it.
In Tomcat 3.x, by default the servlet invoker is enabled. Just place the servlets inside /servlet/ directory and access it by using a fully qualified name like http://[domain]:[port]/[context]/servlet/[servlet.
This mapping is available in web application descriptor (web.xml), located under $TOMCAT_HOME/conf.
/servlet/ is removed from Servlet 2.3 specifications.
In Tomcat 4.x, by defaul the servlet invoker id disabled. The <servlet-mapping> tag is commented inside the default web application descriptor (web.xml), located under $CATALINA_HOME/conf. To enable the invoker servlet uncomment the following two blocks.
<!– The mapping for the invoker servlet –>
<!–
<servlet-mapping>
<servlet-name>invoker</servlet-name>
<url-pattern>/servlet/*</url-pattern>
</servlet-mapping>
–>
…
…
<!–
<servlet>
<servlet-name>invoker</servlet-name>
<servlet-class>
org.apache.catalina.servlets.InvokerServlet
</servlet-class>
<init-param>
<param-name>debug</param-name>
<param-value>0</param-value>
</init-param>
<load-on-startup>2</load-on-startup>
</servlet>
–>
Difference between HttpServlet and GenericServlet
Signature: public abstract class GenericServlet extends java.lang.Object implements Servlet, ServletConfig, java.io.Serializable
- GenericServlet defines a generic, protocol-independent servlet.
- GenericServlet gives a blueprint and makes writing servlet easier.
- GenericServlet provides simple versions of the lifecycle methods init and destroy and of the methods in the ServletConfig interface.
- GenericServlet implements the log method, declared in the ServletContext interface.
- To write a generic servlet, it is sufficient to override the abstract service method.
Signature: public abstract class HttpServlet extends GenericServlet implements java.io.Serializable
- HttpServlet defines a HTTP protocol specific servlet.
- HttpServlet gives a blueprint for Http servlet and makes writing them easier.
- HttpServlet extends the GenericServlet and hence inherits the properties GenericServlet.
What is preinitialization of a java servlet?
The java servlet container first creates the servlet instance and then executes the init() method. This initialization can be done in three ways. The default way is that, the java servlet is initialized when the servlet is called for the first time. This type of servlet initialization is called lazy loading.
The other way is through the
<load-on-startup>non-zero-integer</load-on-startup>
tag using the deployment descriptor web.xml. This makes the java servlet to be loaded and initialized when the server starts. This process of loading a java servlet before receiving any request is called preloading or preinitialization of a servlet.Servlet are loaded in the order of number(non-zero-integer) specified. That is, lower(example: 1) the load-on-startup value is loaded first and then servlet with higher values are loaded.
Example usage:
<servlet>
<servlet-name>Servlet-URL</servlet-name>
<servlet-class>com.javapapers.Servlet-Class</servlet-class>
<load-on-startup>2</load-on-startup>
</servlet>
Difference between ServletConfig and ServletContext
- Signature: public interface ServletConfig
ServletConfig is implemented by the servlet container to initialize a single servlet using init(). That is, you can pass initialization parameters to the servlet using the web.xml deployment descriptor. For understanding, this is similar to a constructor in a java class.
<servlet>
<servlet-name>ServletConfigTest</servlet-name>
<servlet-class>com.javapapers.ServletConfigTest</servlet-class>
<init-param>
<param-name>topic</param-name>
<param-value>Difference between ServletConfig and ServletContext</param-value>
</init-param>
</servlet>
- Signature: public interface ServletContext
ServletContext is implemented by the servlet container for all servlet to communicate with its servlet container, for example, to get the MIME type of a file, to get dispatch requests, or to write to a log file. That is to get detail about its execution environment. It is applicable only within a single Java Virtual Machine. If a web applicationa is distributed between multiple JVM this will not work. For understanding, this is like a application global variable mechanism for a single web application deployed in only one JVM.
Example code:
<context-param>
<param-name>globalVariable</param-name>
<param-value>javapapers.com</param-value>
</context-param>
Difference between ServletRequest.getRequestDispatcher and ServletContext.getRequestDispatcher
- request.getRequestDispatcher(“url”) means the dispatch is relative to the current HTTP request.
Example code:RequestDispatcher reqDispObj = request.getRequestDispatcher("/home.jsp");
- getServletContext().getRequestDispatcher(“url”) means the dispatch is relative to the root of the ServletContext.
Example code:RequestDispatcher reqDispObj = getServletContext().getRequestDispatcher("/ContextRoot/home.jsp");
ServletRequest vs ServletResponse
- Signature: public interface ServletRequest
Blueprint of an object to provide client request information to a servlet. The servlet container creates a ServletRequest object and sends it as an argument to the servlet’s service method. - Signature: public interface ServletResponse
Blueprint of an object to assist a servlet in sending a response to the client. The servlet container creates a ServletResponse object and passes it as an argument to the servlet’s service method. Data that needs to be sent to the client will be put inside the ServletResponse object. To send binary data back to the client in a MIME body response, use the ServletOutputStream from the ServletResponse object by calling the getOutputStream() method. To send character data to the client, the PrintWriter object returned by getWriter() should be used.
Why not declare a constructor in servlet?
Then why is it not customary to declare a constructor in a servlet? Because the init() method is used to perform servlet initialization. In JDK 1.0 (servlet were written in this version), constructors for dynamically loaded Java classes such as servlets cannot accept arguments. Therefore init() was used to initialize by passing the implemented object of ServletConfig interface and other needed parameters.
Also, Java constructors cannot be declared in interfaces. So, javax.servlet.Servlet interface cannot have a constructor that accepts a ServletConfig parameter. To overcome this, init() method is used for initialization instead of declaring a constructor.
Servlet Life Cycle
public void init(ServletConfig config) throws ServletException
public void service(ServletRequest req, ServletResponse res) throws ServletException, java.io.IOException
public void destroy()
-
Creation and initialization
The container first creates the servlet instance and then executes the init() method.
init() can be called only once in its life cycle by the following ways:
a) Through the ‘load-on-startup’ tag using the web.xml. This makes the servlet to be loaded and initialized when the server starts.
b) For the first time only in its life cycle, just before the service() is invoked.
c) Server administrator can request for the initialization of a servlet directly. -
Execution of service
Whenever a client requests for the servlet, everytime the service() method is invoked during its life cycle. From service() then it is branched to the doGet() or doXx..() methods for a HttpServlet. The service() method should contain the code that serves the Servlet purpose. -
Destroy the servlet
destroy() method is invoked first, then Servlet is removed from the container and then eventually garbage collected. destroy() method generally contains code to free any resources like jdbc connection that will not be garbage collected.
A filter is used to dynamically intercept request and response objects and change or use the data present in them. Filters should be configured in the web deployment descriptor. Filters can perform essential functions like authentication blocking, logging, content display style conversion, etc.What is a filter?
0 comments:
Post a Comment