To extend or modify the behaviour of ‘an instance’ at runtime decorator
design pattern is used. Inheritance is used to extend the abilities of ‘a class’. Unlike inheritance, you can choose any single object of a class and
modify its behaviour leaving the other instances unmodified.
In implementing the decorator pattern you construct a wrapper around an object by extending its behavior. The wrapper will do its job before or after and delegate the call to the wrapped instance.
Design of decorator pattern
You start with an
interface which creates a blue print for the class which will have decorators. Then implement that interface with basic functionalities. Till now we have got an interface and an implementation concrete class. Create an
abstract class that contains (
aggregation relationship) an attribute type of the interface. The constructor of this class assigns the interface type instance to that attribute. This class is the decorator base class. Now you can extend this class and create as many concrete decorator classes. The concrete decorator class will add its own methods. After / before executing its own method the concrete decorator will call the base instance’s method. Key to this decorator design pattern is the binding of method and the base instance happens at runtime based on the object
passed as parameter to the constructor. Thus dynamically customizing the behavior of that specific instance alone.
Decorator Design Pattern – UML Diagram
Implementation of decorator pattern
Following given example is an implementation of decorator design pattern. Icecream is a classic example for decorator design pattern. You create a basic icecream and then add toppings to it as you prefer. The added toppings change the taste of the basic icecream. You can add as many topping as you want. This sample scenario is implemented below.
package com.javapapers.sample.designpattern; |
public interface Icecream { |
public String makeIcecream(); |
The above is an interface depicting an icecream. I have kept things as simple as possible so that the focus will be on understanding the design pattern. Following class is a concrete implementation of this interface. This is the base class on which the decorators will be added.
package com.javapapers.sample.designpattern; |
public class SimpleIcecream implements Icecream { |
public String makeIcecream() { |
Following class is the decorator class. It is the core of the decorator design pattern. It contains an attribute for the type of interface. Instance is assigned dynamically at the creation of decorator using its constructor. Once assigned that instance method will be invoked.
package com.javapapers.sample.designpattern; |
abstract class IcecreamDecorator implements Icecream { |
protected Icecream specialIcecream; |
public IcecreamDecorator(Icecream specialIcecream) { |
this .specialIcecream = specialIcecream; |
public String makeIcecream() { |
return specialIcecream.makeIcecream(); |
Following two classes are similar. These are two decorators, concrete class implementing the abstract decorator. When the decorator is created the base instance is passed using the constructor and is assigned to the super class. In the makeIcecream method we call the base method followed by its own method addNuts(). This addNuts() extends the behavior by adding its own steps.
package com.javapapers.sample.designpattern; |
public class NuttyDecorator extends IcecreamDecorator { |
public NuttyDecorator(Icecream specialIcecream) { |
public String makeIcecream() { |
return specialIcecream.makeIcecream() + addNuts(); |
private String addNuts() { |
package com.javapapers.sample.designpattern; |
public class HoneyDecorator extends IcecreamDecorator { |
public HoneyDecorator(Icecream specialIcecream) { |
public String makeIcecream() { |
return specialIcecream.makeIcecream() + addHoney(); |
private String addHoney() { |
Execution of the decorator pattern
I have created a simple icecream and decorated that with nuts and on top of it with honey. We can use as many decorators in any order we want. This excellent flexibility and changing the behaviour of an instance of our choice at runtime is the main advantage of the decorator design pattern.
package com.javapapers.sample.designpattern; |
public class TestDecorator { |
public static void main(String args[]) { |
Icecream icecream = new HoneyDecorator( new NuttyDecorator( new SimpleIcecream())); |
System.out.println(icecream.makeIcecream()); |
Output
Base Icecream + cruncy nuts + sweet honey |
Decorator Design Pattern in java API
java.io.BufferedReader;
java.io.FileReader;
java.io.Reader;
The above readers of java API are designed using decorator design pattern.
An adapter helps two incompatible interfaces to work together. This is the real world definition for an adapter. Adapter design pattern is used when you want two different classes with incompatible interfaces to work together. The name says it all. Interfaces may be incompatible but the inner functionality should suit the need.
In real world the easy and simple example that comes to mind for an adapter is the travel power adapter. American socket and plug are different from British. Their
interface are not compatible with one another. British plugs are cylindrical and American plugs are recangularish. You can use an adapter in between to fit an American (rectangular) plug in British (cylindrical) socket assuming voltage requirements are met with.
How to implement adapter design pattern?
Adapter
design pattern can be implemented in two ways. One using the inheritance method and second using the
composition method. Just the implementation methodology is different but the purpose and solution is same.
Adapter implementation using inheritance
When a class with incompatible method needs to be used with another class you can use inheritance to create an adapter class. The adapter class which is inherited will have new compatible methods. Using those new methods from the adapter the core function of the base class will be accessed. This is called “
is-a” relationship. The same real world example is implemented using java as below. Dont worry too much about logic, following example source code attempts to explain adapter design pattern and the goal is simplicity.
public class CylindricalSocket { |
public String supply(String cylinStem1, String cylinStem1) { |
System.out.println( "Power power power..." ); |
public class RectangularAdapter extends CylindricalSocket { |
public String adapt(String rectaStem1, Sting rectaStem2) { |
String cylinStem1 = rectaStem1; |
String cylinStem2 = rectaStem2; |
return supply(cylinStem1, cylinStem2); |
public class RectangularPlug { |
private String rectaStem1; |
private String rectaStem2; |
RectangulrAdapter adapter = new RectangulrAdapter(); |
String power = adapter.adapt(rectaStem1, rectaStem2); |
System.out.println(power); |
Adapter implementation using composition
The above implementation can also be done using composition. Instead of inheriting the base class create adapter by having the base class as attribute inside the adapter. You can access all the methods by having it as an attribute. This is nothing but “
has-a” relationship. Following example illustrates this approach. Difference is only in the adapter class and other two classes are same. In most scenarios, prefer composition over inheritance. Using composition you can change the behaviour of class easily if needed. It enables the usage of tools like dependency injection.
public class CylindricalSocket { |
public String supply(String cylinStem1, String cylinStem1) { |
System.out.println( "Power power power..." ); |
public class RectangularAdapter { |
private CylindricalSocket socket; |
public String adapt(String rectaStem1, Sting rectaStem2) { |
socket = new CylindricalSocket(); |
String cylinStem1 = rectaStem1; |
String cylinStem2 = rectaStem2; |
return socket.supply(cylinStem1, cylinStem2); |
public class RectangularPlug { |
private String rectaStem1; |
private String rectaStem2; |
RectangulrAdapter adapter = new RectangulrAdapter(); |
String power = adapter.adapt(rectaStem1, rectaStem2); |
System.out.println(power); |
Adapter design pattern in java API
java.io.InputStreamReader(InputStream)
java.io.OutputStreamWriter(OutputStream)
Singleton design pattern is the first
design pattern I learned (many years back). In early days when someone asks me, “do you know any design pattern?” I quickly and promptly answer “I know singleton design pattern” and the question follows, “do you know anything other than singleton” and I stand stumped!
A java beginner will know about singleton design pattern. At least he will think that he knows singleton pattern. The definition is even easier than Newton’s third law. Then what is special about the singleton pattern. Is it so simple and straightforward, does it even deserve an article? Do you believe that you know 100% about singleton design pattern? If you believe so and you are a beginner read through the end, there are surprises for you.
There are only two points in the definition of a singleton design pattern,
- there should be only one instance allowed for a class and
- we should allow global point of access to that single instance.
GOF says, “Ensure a class has only one instance, and provide a global point of access to it. [GoF, p127]“.
The key is not the problem and definition. In singleton pattern, trickier part is implementation and management of that single instance. Two points looks very simple, is it so difficult to implement it. Yes it is very difficult to ensure “single instance” rule, given the flexibility of the APIs and many flexible ways available to access an instance. Implementation is very specific to the language you are using. So the security of the single instance is specific to the language used.
Strategy for Singleton instance creation
We suppress the constructor and don’t allow even a single instance for the class. But we declare an attribute for that same class inside and create instance for that and return it.
Factory design pattern can be used to create the singleton instance.
package com.javapapers.sample.designpattern; |
private static Singleton singleInstance; |
public static Singleton getSingleInstance() { |
if (singleInstance == null ) { |
synchronized (Singleton. class ) { |
if (singleInstance == null ) { |
singleInstance = new Singleton(); |
You need to be careful with multiple threads. If you don’t synchronize the method which is going to return the instance then, there is a possibility of allowing multiple instances in a multi-threaded scenario. Do the synchronization at block level considering the performance issues. In the above example for singleton pattern, you can see that it is threadsafe.
Early and lazy instantiation in singleton pattern
The above example code is a sample for lazy instantiation for singleton design pattern. The single instance will be created at the time of first call of the getSingleInstance() method. We can also implement the same singleton design pattern in a simpler way but that would instantiate the single instance early at the time of loading the class. Following example code describes how you can instantiate early. It also takes care of the multithreading scenario.
package com.javapapers.sample.designpattern; |
private static Singleton singleInstance = new Singleton(); |
public static Singleton getSingleInstance() { |
Singleton and Serialization
Using
serialization, single instance contract of the singleton pattern can be violated. You can serialize and de-serialize and get a new instance of the same singleton class. Using java api, you can implement the below method and override the instance read from the stream. So that you can always ensure that you have single instance.
ANY-ACCESS-MODIFIER Object readResolve() throws ObjectStreamException; |
This article is an attempt to explain the basics on singleton design pattern. If you want more insight on singleton refer this technical article “
When is a Singleton not a Singleton?” and its references.
Usage of Singleton Patter in Java API
java.lang.Runtime#getRuntime() java.awt.Desktop#getDesktop()
When creating an object is time consuming and a costly affair and you already have a most similar object instance in hand, then you go for prototype pattern. Instead of going through a time consuming process to create a complex object, just copy the existing similar object and modify it according to your needs.
Its a simple and straight forward design pattern. Nothing much hidden beneath it. If you don’t have much experience with enterprise grade huge application, you may not have experience in creating a complex / time consuming instance. All you might have done is use the new operator or inject and instantiate.
If you are a beginner you might be wondering, why all the fuss about prototye design pattern and do we really need this design pattern? Just ignore, all the big guys requires it. For you, just understand the pattern and sleep over it. You may require it one day in future.
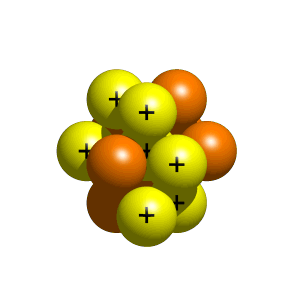
Prototype pattern may look similar to
builder design pattern. There is a huge difference to it. If you remember, “the same construction process can create different representations” is the key in builder pattern. But not in the case of prototype pattern.
So, how to implement the prototype design pattern? You just have to copy the existing instance in hand. When you say copy in java, immediately cloning comes into picture. Thats why when you read about prototype pattern, all the literature invariably refers java cloning.
Simple way is, clone the existing instance in hand and then make the required update to the cloned instance so that you will get the object you need. Other way is, tweak the cloning method itself to suit your new object creation need. Therefore whenever you clone that object you will directly get the new object of desire without modifying the created object explicitly.
The prototype design pattern mandates that the instance which you are going to copy should provide the copying feature. It should not be done by an external utility or provider.
But the above, other way comes with a caution. If somebody who is not aware of your tweaking the clone business logic uses it, he will be in issue. Since what he has in hand is not the exact clone. You can go for a custom method which calls the clone internally and then modifies it according to the need. Which will be a better approach.
Always remember while using clone to copy, whether you need a
shallow copy or deep copy. Decide based on your business needs. If you need a deep copy, you can use serialization as a hack to get the deep copy done. Using clone to copy is entirey a design decision while implementing the prototype design pattern. Clone is not a mandatory choice for prototype pattern.
In prototype pattern, you should always make sure that you are well knowledgeable about the data of the object that is to be cloned. Also make sure that instance allows you to make changes to the data. If not, after cloning you will not be able to make required changes to get the new required object.
Following sample java source code demonstrates the prototype pattern. I have a basic bike in hand with four gears. When I want to make a different object, an advance bike with six gears I copy the existing instance. Then make necessary modifications to the copied instance. Thus the prototype pattern is implemented. Example source code is just to demonstrate the design pattern, please don’t read too much out of it. I wanted to make things as simple as possible.
Sample Java Source Code for Prototype Design Pattern
package com.javapapers.sample.designpattern.prototype; |
class Bike implements Cloneable { |
public void makeAdvanced() { |
public String getModel(){ |
public Bike makeJaguar(Bike basicBike) { |
basicBike.makeAdvanced(); |
public static void main(String args[]){ |
Bike basicBike = bike.clone(); |
Workshop workShop = new Workshop(); |
Bike advancedBike = workShop.makeJaguar(basicBike); |
System.out.println( "Prototype Design Pattern: " +advancedBike.getModel()); |
}
Builder pattern is used to construct a complex object step by step and the final step will return the object. The process of constructing an object should be generic so that it can be used to create different representations of the same object.
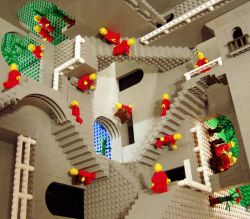
Complex Object Construction
For example, you can consider construction of a home. Home is the final end product (object) that is to be returned as the output of the construction process. It will have many steps, like basement construction, wall construction and so on roof construction. Finally the whole home object is returned. Here using the same process you can build houses with different properties.
GOF says,
“Separate the construction of a complex object from its representation so that the same construction process can create different representations” [GoF 94]
What is the difference between abstract factory and builder pattern?
Abstract factory may also be used to construct a complex object, then what is the difference with builder pattern? In builder pattern emphasis is on ‘step by step’. Builder pattern will have many number of small steps. Those every steps will have small units of logic enclosed in it. There will also be a sequence involved. It will start from step 1 and will go on upto step n and the final step is returning the object. In these steps, every step will add some value in construction of the object. That is you can imagine that the object grows stage by stage. Builder will return the object in last step. But in abstract factory how complex the built object might be, it will not have step by step object construction.
Sample builder design pattern implementation in Java API
DocumentBuilderFactory , StringBuffer, StringBuilder are some examples of builder pattern usage in java API.
Sample Java Source Code for Builder Pattern
Following is the interface, that will be returned as the product from the builder.
package com.javapapers.sample.designpattern.builder; |
public interface HousePlan { |
public void setBasement(String basement); |
public void setStructure(String structure); |
public void setRoof(String roof); |
public void setInterior(String interior); |
Following is the interface for which the factory implementation should be done. Inturn all abstract factory will return this type.
package com.javapapers.sample.designpattern.abstractfactory; |
public interface AnimalFactory { |
public Animal createAnimal(); |
Concrete class for the above interface. The builder constructs an implementation for the following class.
package com.javapapers.sample.designpattern.builder; |
public class House implements HousePlan { |
private String structure; |
public void setBasement(String basement) { |
this .basement = basement; |
public void setStructure(String structure) { |
this .structure = structure; |
public void setRoof(String roof) { |
public void setInterior(String interior) { |
this .interior = interior; |
Builder interface. We will have multiple different implementation of this interface in order to facilitate, the same construction process to create different representations.
package com.javapapers.sample.designpattern.builder; |
public interface HouseBuilder { |
public void buildBasement(); |
public void buildStructure(); |
public void buildInterior(); |
First implementation of a builder.
package com.javapapers.sample.designpattern.builder; |
public class IglooHouseBuilder implements HouseBuilder { |
public IglooHouseBuilder() { |
this .house = new House(); |
public void buildBasement() { |
house.setBasement( "Ice Bars" ); |
public void buildStructure() { |
house.setStructure( "Ice Blocks" ); |
public void buildInterior() { |
house.setInterior( "Ice Carvings" ); |
public void bulidRoof() { |
house.setRoof( "Ice Dome" ); |
public House getHouse() { |
Second implementation of a builder. Tipi is a type of eskimo house.
package com.javapapers.sample.designpattern.builder; |
public class TipiHouseBuilder implements HouseBuilder { |
public TipiHouseBuilder() { |
this .house = new House(); |
public void buildBasement() { |
house.setBasement( "Wooden Poles" ); |
public void buildStructure() { |
house.setStructure( "Wood and Ice" ); |
public void buildInterior() { |
house.setInterior( "Fire Wood" ); |
public void bulidRoof() { |
house.setRoof( "Wood, caribou and seal skins" ); |
public House getHouse() { |
Following class constructs the house and most importantly, this maintains the building sequence of object.
package com.javapapers.sample.designpattern.builder; |
public class CivilEngineer { |
private HouseBuilder houseBuilder; |
public CivilEngineer(HouseBuilder houseBuilder){ |
this .houseBuilder = houseBuilder; |
public House getHouse() { |
return this .houseBuilder.getHouse(); |
public void constructHouse() { |
this .houseBuilder.buildBasement(); |
this .houseBuilder.buildStructure(); |
this .houseBuilder.bulidRoof(); |
this .houseBuilder.buildInterior(); |
Testing the sample builder design pattern.
package com.javapapers.sample.designpattern.builder; |
public class BuilderSample { |
public static void main(String[] args) { |
HouseBuilder iglooBuilder = new IglooHouseBuilder(); |
CivilEngineer engineer = new CivilEngineer(iglooBuilder); |
engineer.constructHouse(); |
House house = engineer.getHouse(); |
System.out.println( "Builder constructed: " +house); |
Output of the above sample program for builder pattern
Builder constructed: com.javapapers.sample.designpattern.builder.House@7d772e
Factory of factories. To keep things simple you can understand it like, you have a set of ‘related’
factory method design pattern. Then you will put all those set of simple factories inside a factory pattern. So in turn you need not be aware of the final concrete class that will be instantiated. You can program for the interface using the top factory.
There is also a view that abstract factory is ‘also’ implemented using prototype instead of factory methords pattern. Beginners for now please don’t yourself with that. Just go with factory methods pattern.
As there is a word ‘abstract’ in the pattern name don’t mistake and confuse it with java ‘abstract’ keyword. It is not related to that. This abstract is from object oriented programming paradim.
Sample abstract factory design pattern implementation in Java API
XML API implements abstract factory. There is a class name
SchemaFactory. This acts as a factory and supports implemenation of multiple schemas using abstract factory design pattern.
Sample Java Source Code for Factory Method Design Pattern
Following is the interface, that will be returned as the final end product from the factories.
package com.javapapers.sample.designpattern.abstractfactory; |
public interface Animal { |
Following is the interface for which the factory implementation should be done. Inturn all abstract factory will return this type.
package com.javapapers.sample.designpattern.abstractfactory; |
public interface AnimalFactory { |
public Animal createAnimal(); |
One of the factory from a predefined set which will instantiate the above interface.
package com.javapapers.sample.designpattern.abstractfactory; |
public class SeaFactory implements AnimalFactory { |
public Animal createAnimal() { |
Second factory from a predefined set which will instantiate the Animal interface.
package com.javapapers.sample.designpattern.abstractfactory; |
public class LandFactory implements AnimalFactory { |
public Animal createAnimal() { |
Implementation of an Animal. This class is grouped with the first abstract factory.
package com.javapapers.sample.designpattern.abstractfactory; |
public class Shark implements Animal { |
System.out.println( "I breathe in water! He he!" ); |
Implementation of an Animal. This class is grouped with the second abstract factory.
package com.javapapers.sample.designpattern.abstractfactory; |
public class Elephant implements Animal { |
System.out.println( "I breathe with my lungs. Its easy!" ); |
Following class consumes the abstract factory.
package com.javapapers.sample.designpattern.abstractfactory; |
public class Wonderland { |
public Wonderland(AnimalFactory factory) { |
Animal animal = factory.createAnimal(); |
Testing the abstract factory design pattern.
package com.javapapers.sample.designpattern.abstractfactory; |
public class SampleAbstractFactory { |
public static void main(String args[]){ |
new Wonderland(createAnimalFactory( "water" )); |
public static AnimalFactory createAnimalFactory(String type){ |
return new LandFactory(); |
Output of the above sample program for abstract factory pattern
I breathe in water! He he!
05/11/2009
A factory method pattern is a creational pattern. It is used to instantiate an object from one among a set of classes based on a logic.
Assume that you have a set of classes which extends a common super class or interface. Now you will create a concrete class with a method which accepts one or more arguments. This method is our factory method. What it does is, based on the arguments passed factory method does logical operations and decides on which sub class to instantiate. This factory method will have the super class as its return type. So that, you can program for the interface and not for the implementation. This is all about factory method design pattern.
Sample factory method design pattern implementation in Java API
For a reference of how the factory method design pattern is implemented in Java, you can have a look at SAXParserFactory. It is a factory class which can be used to intantiate SAX based parsers to pares XML. The method
newInstance is the factory method which instantiates the sax parsers based on some predefined logic.
Block diagram for The Design Pattern
Sample Java Source Code for Factory Method Design Pattern
Based on comments received from users, I try to keep my sample java source code as simple as possible for a novice to understand.
Base class:
package com.javapapers.sample.designpattern.factorymethod; |
First subclass:
package com.javapapers.sample.designpattern.factorymethod; |
public class Dog implements Pet { |
Second subclass:
package com.javapapers.sample.designpattern.factorymethod; |
public class Duck implements Pet { |
Factory class:
package com.javapapers.sample.designpattern.factorymethod; |
public class PetFactory { |
public Pet getPet(String petType) { |
if ( "bark" .equals(petType)) |
else if ( "quack" .equals(petType)) |
Using the factory method to instantiate
package com.javapapers.sample.designpattern.factorymethod; |
public class SampleFactoryMethod { |
public static void main(String args[]){ |
PetFactory petFactory = new PetFactory(); |
Pet pet = petFactory.getPet( "bark" ); |
System.out.println(pet.speak()); |
Output of the above sample program for Factory Method Pattern
Introduction To Design Patterns
Pattern is a defined, used and tested solution for a know problem. Design patterns is all about re-use. Software design patterns evolved as a subject of study only when object oriented programming started becoming popular. OOPS and design patterns became inseparable.
In OOPS, we should have well defined boundaries for objects. That is every object should have its roles and responsibilities well defined. Then at next level, we should have a clear interaction plan between objects. If you design a OO software with the above principle, then by default you will be following some of the already defined design patterns.
A formal definition for design patterns, “A design pattern addresses a recurring design problem that arises in specific design situations and presents a solution to it” (Buschmann, et. al. 1996)
Java widely uses design patterns in its APIs. It started as early as Java 1.2 in java foundation classes. By then you can see the widespread use of commonly know design patterns in collections framework and IO packages. When I say commonly known design patterns, I mention about the set of 23 design patterns by Gang of Four (GOF). Gamma, Helm, Johnson and Vlissides known as Gang of Four (GOF) published a book “Design Patterns — Elements of Reusable Software” (1995) based on their series of technical meetings. It is one of the best seller in computer science books till date.
In China gang of four means different set of people. Jiang Qing (Mao Zedong’s fourth wife), Zhang Chunqiao, Yao Wenyuan, and Wang Hongwen were very popular leaders of cultural revolution. They almost seized power after Mao Zedong’s death. But they were finally arrested and imprisoned for life.
Our GOF divided the 23 design patterns into three types creational design patterns, structural design patterns and behavioral design patterns.
Creational design patterns can be used to instantiate objects. Instead of instantiating objects directly, depending on scenario either X or Y object can be instantiated. This will give flexibility for instantiation in high complex business logic situations.
Structural design patterns can be used to organize your program into groups. This segregation will provide you clarity and will enable you for easier maintainability.
Behavioral design patterns can be used to define the communication and control flow between objects.
Following this post, I have planned to write a series of article on these design patterns with java source code examples and UML diagrams. Looking forward to your comments.
0 comments:
Post a Comment